Create WCF service and use it to retreive data from Dynamics CRM
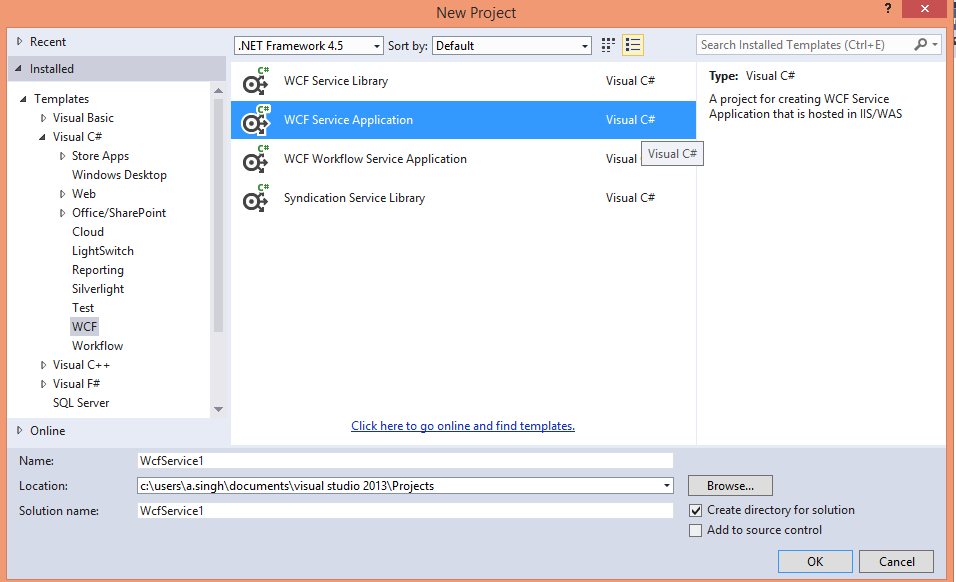
1. Open Visual Studio and Select WCF service application. 2. Open IService1.cs and add following code:: namespace WcfServiceCRM { // NOTE: You can use the "Rename" command on the "Refactor" menu to change the interface name "IService1" in both code and config file together. [ServiceContract] public interface IService1 { [OperationContract] Account[] getAccountDetails(); // TODO: Add your service operations here } // Use a data contract as illustrated in the sample below to add composite types to service operations. [DataContract] public class Account { string fullname; string companyname; string telephone; [DataMember] public string FullName { get { return fullname; } set { fullname = value; } } [DataMember] public string CompanyName { get { r